Image Search Source Code Link:
Today we have a fun little project. We are going to do Reverse Image Search.
Workflow for the Image Search:
- Choose an image
- Initialize number and color, of segments for that image
- Run KMeans to segment that image into regions
- Find the most similar image based on the regions extracted and processed from both images
Introduction to Reverse Image Search
This project is all about comparing two images and measure their similarity. There are plenty of ways how you can calculate that percentage, but we will do it using K-Means and image segmentation. The idea is to divide the image into regions, and base our similarity percentage on that. Google has implemented their own way of Reverse Image Search algorithm and you can check it our here.
Choose an image, and drop it down or upload it to images.google.com. Google will find the most similar/exact match to your image search query.
Example:
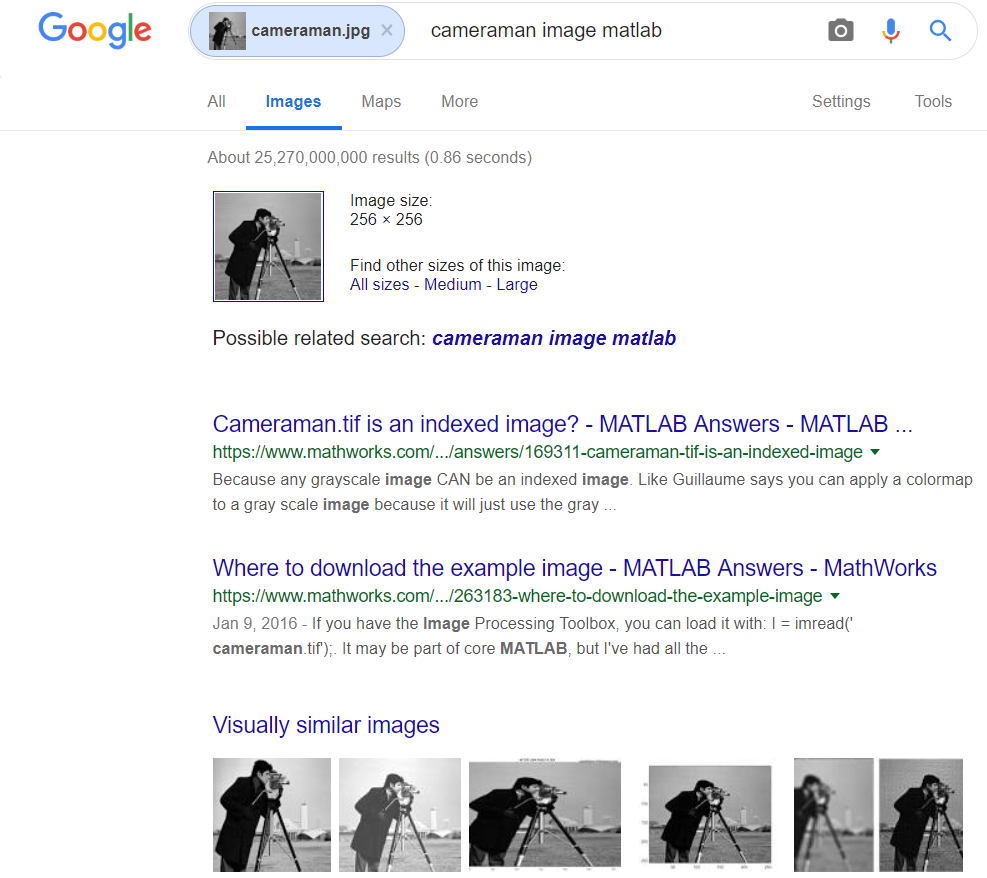
Like I mentioned already, for now we will be using a very basic concept to calculate the similarity, but this is only part one. In the next part we will look at the context of the image rather then just measuring region similarity. Doing an image searching based on the context of the image will yield even better results.
Let’s get started…
Choose an image
Since we are about to do an image search, we need to select an image. In the video presentation above I have chosen the famous “Cameraman” image.
For this project we will be using grayscale images. If you wish to search for a RGB image, you can grayscale it, and then continue with the same procedure.
Select number of regions for Image Search
So this part is very simple. In order for K-Means to segment the image into regions, we first assign the number of K (number of regions) for the image. The bigger the K, the more regions should appear.
If you are not familiar with K-Means you can find more articles/projects about it on my blog:
- How K-Means works – take a look at this project in order to see how K-Means algorithm works visually in 2D space
- K-Means Elbow Method – if you are not sure how many centroids are there in the dataset, check out this project to see how to find out the correct number.
- Shape Recognition with K-Means – will show you how to apply K-Means to a share recognition problem
Image Segmentation
Since we have already chosen an image to search. It is time to process the image. Like I said at the beginning. If the image you want to search for is in a RGB format, then make sure you grayscale it first and then proceed with the rest of this tutorial.
K-Means for Image Segmentation
In case you are wondering how you can perform image segmentation using K-Means, you can check the following link:
In the following image I have the results from the image segmentation using two and three regions/centroids for the cameraman image.
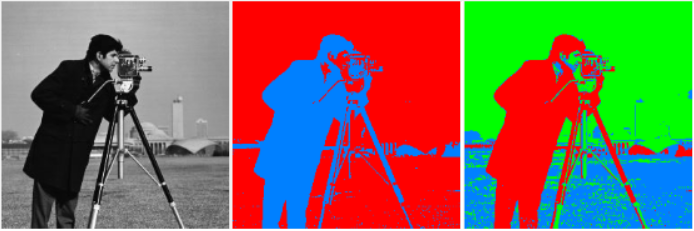
The idea is that a similar image would have similar regions. We will see how we will calculate that in the following part of the tutorial.
Image Search
OK, so now we have segmented our image into regions. We are ready to search.
In the demo application, I have built in option to load images from a selected folder on our PC. The app will calculate the image similarity for all the images in the selected folder. Ultimately it will sort the list based on similarity as shown in the following image.
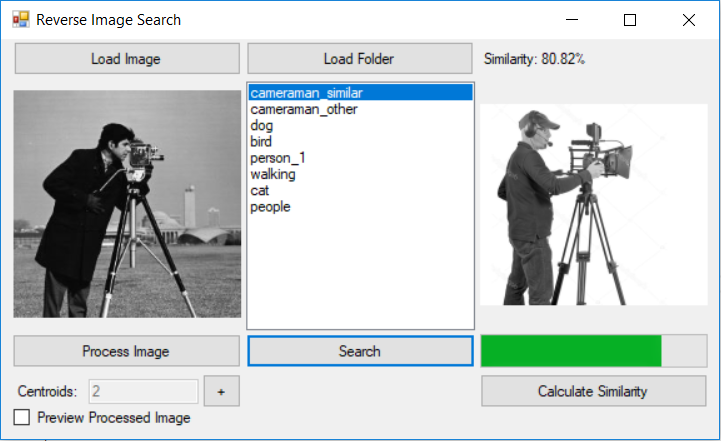
At the moment we are calculating the similarity based on the pixels in a region. Here is a code snapshot:
public double CalculateSimilarity(Bitmap bmpImage1, Bitmap bmpImage2)
{
int correct = 0;
for (int i = 0; i < bmpImage1.Width; i++)
{
for (int j = 0; j < bmpImage1.Height; j++)
{
Color c1 = bmpImage1.GetPixel(i, j);
Color c2 = bmpImage2.GetPixel(i, j);
if (c1.ToArgb() == c2.ToArgb())
correct++;
}
}
int maxPixels = bmpImage1.Width * bmpImage1.Height;
double SimilarityPercent = (100.0 * correct)/maxPixels;
return SimilarityPercent;
}
As you can see from the code, both images are processed. And each processed image is colored in their respective region colors that we have set up at the beginning. All that is left is to count the number of pixels that match (belong to same region), and calculate the percentage.
Final words…
There it is… Very simple.
Next time we will try to extract regions and compare the similarity based on the context of the image.
If You have ideas on how to improve the current solution, please write them down in the comment section.
Vern
June 10, 2021 at 11:23 pmYour download link does not work.
Ertan KILIC
January 30, 2024 at 6:07 pmHello,
I want to search for bmp images and I get this error.
System.ArgumentOutOfRangeException: ‘Parameter must be positive and less than Width.
Parameter name: x’
best gold ira
February 5, 2024 at 3:00 amI think this is a bit out of touch-you should be clearer?
najlepszy sklep
February 13, 2024 at 8:53 pmI think the admin of this web page is really working hard in support of his
website, since here every material is quality based data.
e-commerce
February 14, 2024 at 8:28 amI am in fact thankful to the holder of this website who has shared this wonderful paragraph at
at this time.!
relax sugar free cbd gummies
February 24, 2024 at 8:46 amdelta 8 in tennessee
sklep online
March 14, 2024 at 7:23 pmI really like it when folks come together and share thoughts.
Great blog, continue the good work! I saw similar here: Sklep online
dobry sklep
March 17, 2024 at 9:49 amall the time i used to read smaller content that also clear their motive, and that is also happening with this post which I am reading here.
I saw similar here: Sklep internetowy
pink meta moon
March 22, 2024 at 8:51 pmHi, It has come to our attention that you are using our client’s photographs on your site without a valid licence. We have already posted out all supporting documents to the address of your office. Please confirm once you have received them. In the meantime, we would like to invite you to settle this dispute by making the below payment of £500. Visual Rights Group Ltd, KBC Bank London, IBAN: GB39 KRED 1654 8703, 1135 11, Account Number: 03113511, Sort Code: 16-54-87 Once you have made the payment, please email us with your payment reference number. Please note that a failure to settle at this stage will only accrue greater costs once the matter is referred to court. I thank you for your cooperation and look forward to your reply. Yours sincerely, Visual Rights Group Ltd, Company No. 11747843, Polhill Business Centre, London Road, Polhill, TN14 7AA, Registered Address: 42-44 Clarendon Road, Watford WD17 1JJ
sklep online
March 24, 2024 at 12:42 pmHowdy! Do you know if they make any plugins to help with SEO?
I’m trying to get my blog to rank for some targeted keywords
but I’m not seeing very good gains. If you know of any please share.
Kudos! You can read similar art here: E-commerce
Analytical Agency
March 24, 2024 at 9:00 pmIt’s very interesting! If you need help, look here: ARA Agency
vpn special coupon
March 24, 2024 at 10:12 pmHello, I check your new stuff regularly. Your humoristic
style is witty, keep up the good work!
Here is my site: vpn special coupon
najlepszy sklep
March 28, 2024 at 7:48 amHowdy! Do you know if they make any plugins to assist with Search Engine Optimization? I’m trying to get my
blog to rank for some targeted keywords but I’m not seeing very
good results. If you know of any please share. Kudos! You can read similar article here: Sklep internetowy
click here
March 29, 2024 at 5:57 pmGood information click here
click here
March 29, 2024 at 5:58 pmThanks for sharing such informative information click here
najlepszy sklep
March 29, 2024 at 11:23 pmHello there! Do you know if they make any plugins to assist with Search Engine Optimization? I’m trying to get my blog to
rank for some targeted keywords but I’m not seeing very good results.
If you know of any please share. Thank you! You can read
similar article here: Dobry sklep
vpn code 2024
March 30, 2024 at 9:52 pmHello there, I found your website by way of Google while looking for a comparable subject, your website
got here up, it appears to be like great.
I’ve bookmarked it in my google bookmarks.
Hello there, just turned into aware of your blog thru
Google, and located that it is truly informative. I am
going to watch out for brussels. I will appreciate for those who continue this in future.
Lots of other people will likely be benefited out of your writing.
Cheers!
my web blog :: vpn code 2024
vpn special coupon
April 2, 2024 at 4:19 amI don’t know whether it’s just me or if perhaps everyone else experiencing issues with your blog.
It seems like some of the written text on your
posts are running off the screen. Can somebody
else please comment and let me know if this is happening
to them as well? This might be a problem with my browser
because I’ve had this happen previously. Thank you
Visit my web blog :: vpn special coupon
vpn code 2024
April 3, 2024 at 8:35 amHowdy just wanted to give you a quick heads up.
The text in your post seem to be running off
the screen in Opera. I’m not sure if this is a formatting issue or something to do with web browser compatibility
but I thought I’d post to let you know. The style and design look great though!
Hope you get the issue resolved soon. Thanks
Here is my web page; vpn code 2024
https://hitman.agency/how-to-auto-approve-list/
April 4, 2024 at 3:44 amHowdy! Do you know if they make any plugins to help with SEO?
I’m trying to get my site to rank for some targeted keywords but
I’m not seeing very good gains. If you know
of any please share. Thank you! You can read similar
article here: Scrapebox AA List
vpn special code
April 5, 2024 at 4:39 amHi there i am kavin, its my first time to commenting anyplace, when i read this piece
of writing i thought i could also make comment due to this sensible paragraph.
My web blog … vpn special code
vpn special code
April 10, 2024 at 7:11 amAfter I initially commented I seem to have clicked the -Notify me when new comments are added- checkbox and from now on each time a comment is added I receive
4 emails with the exact same comment. Perhaps there is a means
you can remove me from that service? Cheers!
My page vpn special code
lunasolix.top
April 15, 2024 at 7:42 pmWow, fantastic blog layout! How long have you ever been blogging
for? you make blogging glance easy. The total look of your website is fantastic, as well as the content
material! You can see similar here sklep internetowy
seo optimization
April 24, 2024 at 8:48 amGood read.. keep writing.. I would like to suggest writer and reader about seo optimization info at https://www.seoexpertservices.co/